CALL executes operations against the query result of each subquery, NOT all the result of a query command. It wraps query commands and the operations in curly braces {}
, passes in the alias that triggers each subquery using a WITH clause and passes out the result of each subquery using a RETURN clause.
Syntax:
CALL {
with <alias_In>
, <alias_In>
, ...
...
return <expression>
as <alias_Out>
, <expression>
as <alias_Out>
, ...
}
Input:
- <alias_In>: The alias to pass into the CALL clause
- <expression>: The return value of the subquery
- <alias_Out>: The alias of return value of the subquery to pass out of the CALL clause, optional when <expression> is alias
find().nodes([1, 5]) as nodes
call {
with nodes
n(nodes).e()[:2].n() as p
skip 1
return p as path
}
return path
In the UQL above, there are 3 p resulted from the 1st subquery and 2 p resulted from the 2nd subquery, one row is skipped for each of these two results:
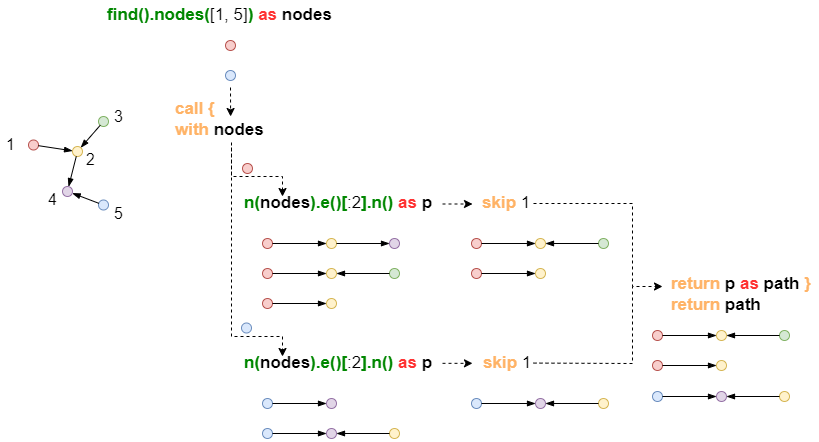
Single Query in Subquery
Example: do not use GROUP BY, find how many cards Customer CU001, CU002, and CU003 hold respectively
uncollect ["CU001", "CU002", "CU003"] as user
call {
with user
n({_id == user}).e({@has}).n({@card} as n)
return count(n) as number
}
return user, number
Analysis: CALL aggregates each user
's n
to get number
, i.e. group all user
s n
based on user
and aggregate all user
s n
in group.
Multiple Queries in Subquery
Example: find 10 transfer edges with an amount higher than 8,000 and rate each edge: if there is transfer between its end-node and bank card CA029 within 2 steps, then add 0.5 for that end-node; the sum of ratings of both end-nodes is the final rating of the edge
n({@card} as n1).re({@transfer.amount > 8000} as e).n({@card} as n2).limit(10)
call {
with n1, n2
optional n(n1 as a1).e({@transfer})[:2].n({_id == "CA029"}).limit(1)
with CASE a1 when 0 then 0 else 0.5 END as c1
optional n(n2 as a2).e({@transfer})[:2].n({_id == "CA029"}).limit(1)
with CASE a2 when 0 then 0 else 0.5 END as c2
return c1+c2 as c
}
return e{*}, c
Analysis: n1
and n2
are the startting-node and ending-node of edge e
, CALL calculates ratings c1
and c2
for n1
and n2
in each row, and sum them as the total rating c
for each e
; the length of c
is the same as the length of n1
, n2
and c
.