Insert operation means to insert one or multiple nodes/edges into the current GraphSet; commands insert()
, insert().overwrite()
and upsert()
all can trigger insert operation. This article introduces the insert operation triggered by command insert()
.
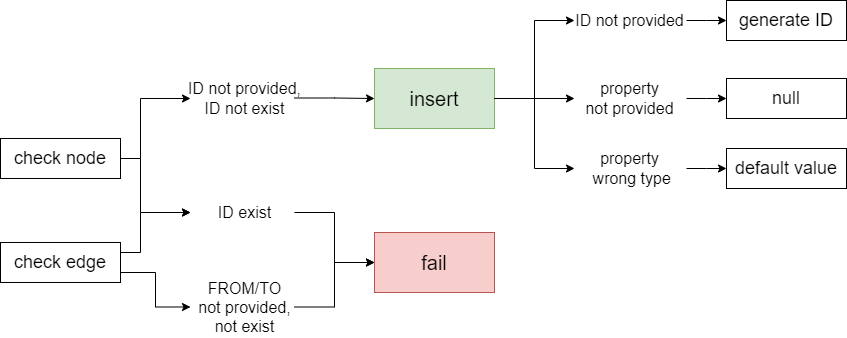
insert()
may trigger:
- an insert operation if:
- ID is not provided
- the provided ID does not exist in the graph
- a failure if:
- the provided ID already exists in the graph
- FROM or TO is not provided when inserting edges
- provided FROM or TO does not exist in the graph
When insert operation is triggered:
- the missing ID will be generated by system
- the missing custom properties will be
null
- the custom properties provided in the wrong data type will be using default value
- "" (string, text)
- 0 (int32, int64, uint32, uint64, float, double, decimal)
- "1970-01-01 08:00:00 +08:00" (timestamp)
- "0000-00-00 00:00:00" (datetime)
- [] (list)
- POINT(0.00 0.00) (point)
It is not suggested to execute insertion operation after streaming return of an algorithm, see details on
stream()
in document Ultipa Graph Analytics & Algorithms - Using Algorithms - Execution Method.
Syntax:
- Statement alias: supported (NODE or EDGE)
// To insert nodes of a certain schema in the current graphset
insert().into(@<schema>)
.nodes([ // Square brackets can be omitted if inserts only one node
{<property1>:<value1>, <property2>:<value2>, ...},
{<property1>:<value1>, <property2>:<value2>, ...},
...
])
// To insert edges of a certain schema in the current graphset, must carry _from and _to, or must carry _from_uuid and _to_uuid
insert().into(@<schema>)
.edges([ // Square brackets can be omitted if inserts only one edge
{<property1>:<value1>, <property2>:<value2>, ...},
{<property1>:<value1>, <property2>:<value2>, ...},
...
])
Example: Insert a node of @default, provide properties 'point' and 'blob'
insert().into(@default).nodes({
point: point({latitude: 132.1, longitude: -1.5}),
blob: castToRaw("32")
})
Analysis: When inserting type piont and blob, refer to related documents for the usage of functions point()
and castToRaw()
.
Sample graph: (to be used for the following examples)
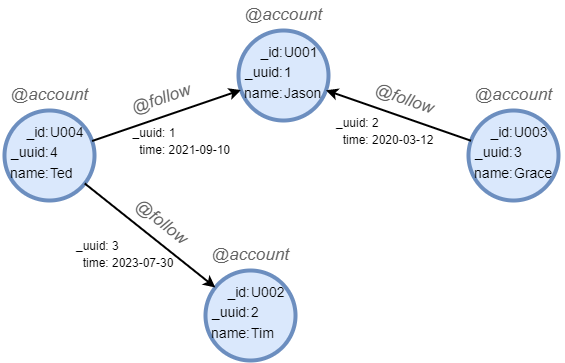
create().node_schema("account").edge_schema("follow")
create().node_property(@account, "name").edge_property(@follow, "time", datetime)
insert().into(@account).nodes([{_id:"U001", _uuid:1, name:"Jason"}, {_id:"U002", _uuid:2, name:"Tim"}, {_id:"U003", _uuid:3, name:"Grace"}, {_id:"U004", _uuid:4, name:"Ted"}])
insert().into(@follow).edges([{_uuid:1, _from_uuid:4, _to_uuid:1, time:"2021-09-10"}, {_uuid:2, _from_uuid:3, _to_uuid:2, time:"2020-03-12"}, {_uuid:3, _from_uuid:4, _to_uuid:2, time:"2023-07-30"}])
Example: Insert two nodes into @account, one does not carry any information, namely, the _id
and _uuid
will be generated and all custom properties are null
; the other carries a nonexistent _id
and all properties in correct data type, in which case only the _uuid
is generated
insert().into(@account).nodes([{}, {_id: "U005", name: "Alice"}]) as nodes
return nodes{*}
| _id | _uuid | name |
|------------------------|-------|--------|
| ULTIPA8000000000000001 | 5 | null |
| U005 | 6 | Alice |
Example: Prompt error when inserting node due to that ID already exist
insert().into(@account).nodes({_id: "U001"}) as nodes
return nodes{*}
Duplicated ids!
Example: Prompt error when inserting edge due to that TO does not exist
insert().into(@follow).edges({_from: "U001", _to: "U010"}) as edges
return edges{*}
The _to: U010 does not exist!