Overview
Graph traversal is a search technique used to visit and explore all the nodes of a graph systematically. The primary goal of graph traversal is to uncover and examine the structure and connections of the graph. There are two common strategies for graph traversal:
- Breadth-First Seach (BFS)
- Depth-First Search (DFS)
The Depth-First Search (DFS) algorithm operates based on the backtracking principle and follows these steps:
- Create a stack (last in, first out) to keep track of visited nodes.
- Start from a selected node, push it into the stack, and mark it as visited.
- Push any unvisited neighbor of the node at the top of the stack into the stack, and mark it as visited. If there are multiple unvisited neighbors, choose one arbitrarily or based on a certain order.
- Repeat step 3 until there are no more unvisited neighbors to push into the stack.
- When there are no new nodes to visit, backtrack to the previous node (the one from which the current node was explored) by popping the top node from the stack.
- Repeat steps 3, 4 and 5 until the stack is empty.
Below is an example of traversing the graph using the DFS approach, starting from node A and assuming to visit neighbors in alphabetical order (A~Z):
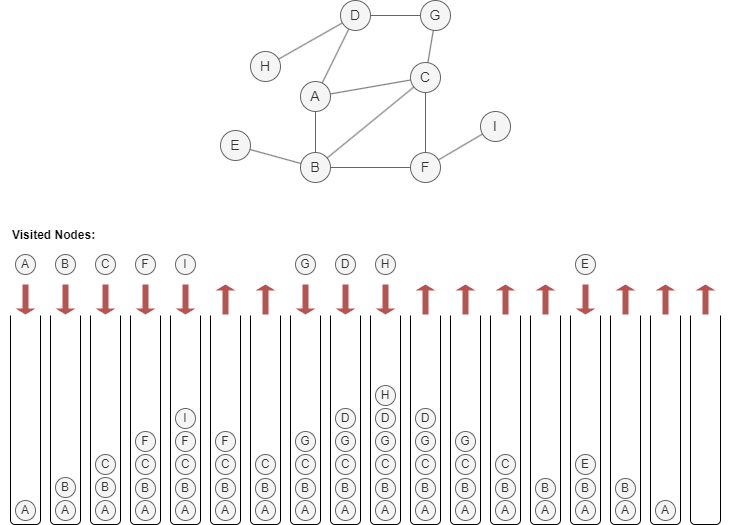
Considerations
- Only nodes that are in the same connected component as the start node can be traversed. Nodes in different connect components will not be included in the traversal results.
Syntax
- Command:
algo(traverse)
- Parameters:
Name |
Type |
Spec |
Default |
Optional |
Description |
---|---|---|---|---|---|
ids / uuids | _id / _uuid |
/ | / | No | ID/UUID of the start node to traverse the graph |
direction | string | in , out |
/ | Yes | Direction of edges when traversing the graph |
traverse_type | string | dfs |
bfs |
No | To traverse the graph in the DFS approach, keep it as dfs |
Examples
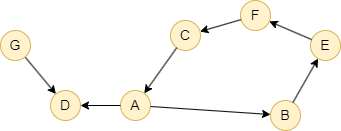
File Writeback
Spec |
Content |
Description |
---|---|---|
filename | _id,_id |
The visited node (toNode), and the node from which it is visited (fromNode) |
algo(traverse).params({
ids: ['B'],
direction: 'in',
traverse_type: 'dfs'
}).write({
file: {
filename: 'result'
}
})
Results: File result
F,C
E,F
C,A
B,B
A,B