Overview
The SET
statement allows you to update or remove properties on nodes and edges. These nodes or edges must first be retrieved using the MATCH
statement.
Note: The schema (label) of a node or edge cannot be modified. Similarly, the values of system properties (_id
, _uuid
, _from
, _to
, _from_uuid
, and _to_uuid
) are immutable.
<set statement> ::=
"SET" <set item> [ { "," <set item> }... ]
<set item> ::=
<set single property> | <set all properties>
<set single property> ::=
<graph element variable reference> "." <property name> "=" <value expression>
<set all properties> ::=
<graph element variable reference> "=" <property specification>
Details
- You can either update individual properties (e.g.,
SET n.age = 12
, which updatesage
to12
and keeps other property values unchanged), or overwrite all properties (e.g.,SET n = {age: 12}
, which updatesage
to12
and removes all other proeprty values).
Example Graph
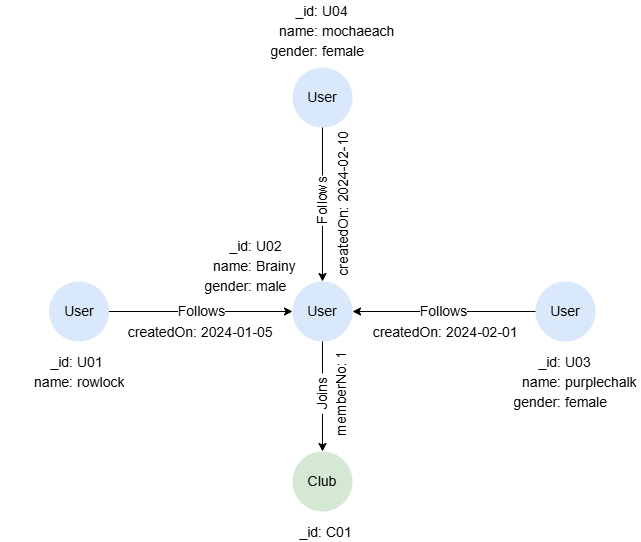
CREATE GRAPH myGraph {
NODE User ({name string, gender string}),
NODE Club (),
EDGE Follows ()-[{createdOn date, weight int32}]->(),
EDGE Joins ()-[{memberNo uint32}]->()
} PARTITION BY HASH(Crc32) SHARDS [1]
INSERT (rowlock:User {_id: "U01", name: "rowlock"}),
(brainy:User {_id: "U02", name: "Brainy", gender: "male"}),
(purplechalk:User {_id: "U03", name: "purplechalk", gender: "female"}),
(mochaeach:User {_id: "U04", name: "mochaeach", gender: "female"}),
(c:Club {_id: "C01"}),
(rowlock)-[:Follows {createdOn: "2024-01-05"}]->(brainy),
(purplechalk)-[:Follows {createdOn: "2024-02-01"}]->(brainy),
(mochaeach)-[:Follows {createdOn: "2024-02-10"}]->(brainy),
(brainy)-[:Joins {memberNo: 1}]->(c)
Updating Properties
To update gender
of the retrieved node n
and createdOn
of the retrieved edge e
:
MATCH (n:User {name: 'rowlock'})-[e:Follows]->(:User {name: 'Brainy'})
SET n.gender = 'male', e.createdOn = '2024-01-07'
RETURN n.gender, e.createdOn
Result:
n.gender | e.createdOn |
---|---|
male | 2024-01-07 |
Removing Properties
To remove the value of gender
of the retrieved node n
:
MATCH (n:User {name: 'mochaeach'})
SET n.gender = null
RETURN n
Result: n
_id | _uuid | schema | values |
---|---|---|---|
U04 | Sys-gen | User | {name: "mochaeach", gender: null} |
Overwriting All Properties
To overwrite all properties of the retrieved node n
, updating the value of name
and removing values of other properties:
MATCH (n:User {name: 'purplechalk'})
SET n = {name: 'MasterSwift'}
RETURN n
Result: n
_id | _uuid | schema | values |
---|---|---|---|
U03 | Sys-gen | User | {name: "MasterSwift", gender: null} |
Removing All Properties
To remove all properties of the retrieved node n
:
MATCH (n:User {name: 'rowlock'})
SET n = {}
RETURN n
Result: n
_id | _uuid | schema | values |
---|---|---|---|
U03 | Sys-gen | User | {name: null, gender: null} |
Updating Properties with CASE
To assign values to weight
of Follows
edges based on their createdOn
values:
MATCH ()-[e:Follows]->()
SET e.weight = CASE
WHEN e.createdOn < '2024-01-31' THEN 10
ELSE 8
END
RETURN e
Result: e
_uuid |
_from |
_to |
_from_uuid |
_to_uuid |
schema |
values |
---|---|---|---|---|---|---|
Sys-gen | U01 | U02 | UUID of U01 | UUID of U02 | Follows | {createdOn: "2024-01-05", weight: 10} |
Sys-gen | U04 | U02 | UUID of U04 | UUID of U02 | Follows | {createdOn: "2024-02-10", weight: 8} |
Sys-gen | U03 | U02 | UUID of U03 | UUID of U02 | Follows | {createdOn: "2024-02-01", weight: 8} |
Mismatched Value Type
If the provided value does not match the property's expected value type and cannot be converted, the property will be assigned its default value based on the property value type.
To update memberNo
(int32
type) of the retrieved edge with a string:
MATCH ()-[e:Joins]->()
SET e.memberNo = 'm2'
RETURN e
Result: e
_uuid |
_from |
_to |
_from_uuid |
_to_uuid |
schema |
values |
---|---|---|---|---|---|---|
Sys-gen | C01 | U02 | UUID of C01 | UUID of U02 | Follows | {memberNo: 0} |