Overview
The insert().into()
clause facilitates the insertion of new nodes or edges within a single schema.
Syntax
// Insert nodes
insert().into(@<schema>).nodes([
{<property1>: <value1>, <property2>: <value2>, ...},
{<property1>: <value1>, <property2>: <value2>, ...}
])
// Insert edges
insert().into(@<schema>).edges([
{<property1>: <value1>, <property2>: <value2>, ...},
{<property1>: <value1>, <property2>: <value2>, ...}
])
- Specify one schema in the
into()
method. - Include one or multiple nodes or edges in the
nodes()
oredges()
method.- Provide key-value pairs of properties for each node or edge enclosed in
{ }
. - If there is only one node or edge, the outer
[ ]
can be omitted.
- Provide key-value pairs of properties for each node or edge enclosed in
- Allow to define an alias for the clause, with the data type being either NODE or EDGE.
Inserting nodes:
- No property is mandatory to be provided.
- If the unique identifier property
_id
or_uuid
is missing, its value will be generated by the system. - The values of missing custom properties will be
null
.
Inserting edges:
- The properties
_from
and_to
(or_from_uuid
and_to_uuid
) are mandatory to be provided to specify the start and end nodes of the edge. Other properties are optional. - If the unique identifier property
_uuid
is missing, its value will be automatically generated by the system. - The values of missing custom properties will be
null
.
Example Graph
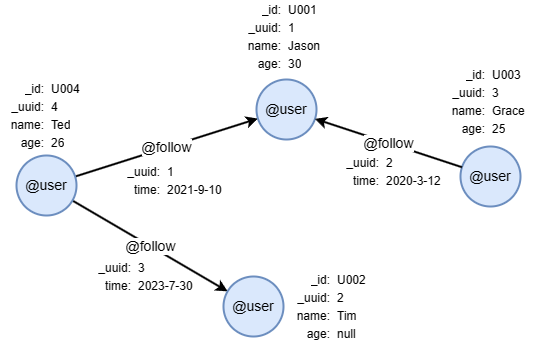
Run these UQLs row by row in an empty graphset to create this graph:
create().node_schema("user").edge_schema("follow")
create().node_property(@user, "name").node_property(@user, "age", int32).edge_property(@follow, "time", datetime)
insert().into(@user).nodes([{_id:"U001", _uuid:1, name:"Jason", age:30}, {_id:"U002", _uuid:2, name:"Tim"}, {_id:"U003", _uuid:3, name:"Grace", age:25}, {_id:"U004", _uuid:4, name:"Ted", age:26}])
insert().into(@follow).edges([{_uuid:1, _from_uuid:4, _to_uuid:1, time:"2021-9-10"}, {_uuid:2, _from_uuid:3, _to_uuid:2, time:"2020-3-12"}, {_uuid:3, _from_uuid:4, _to_uuid:2, time:"2023-7-30"}])
Examples
Insert Single Node
insert().into(@user).nodes({_id: "U005", name: "Alice"})
A new node is inserted with the following properties:
_id | _uuid | name | age |
---|---|---|---|
U005 | 5 | Alice | null |
Insert Single Edge
insert().into(@follow).edges({_from: "U002", _to: "U001", time: "2023-8-9"})
A new edge is inserted with the following properties:
_uuid | _from | _to | _from_uuid |
_to_uuid | time |
---|---|---|---|---|---|
4 | U002 | U001 | 2 | 1 | 2023-08-09 00:00:00 |
Insert Multiple Nodes
insert().into(@user).nodes([
{name: "Lee", age: 12},
{_uuid: 10, name: "Alex"},
{}
])
Three new nodes are inserted with the following properties:
_id |
_uuid | name | age |
---|---|---|---|
ULTIPA8000000000000009 | 6 | Lee | 12 |
ULTIPA8000000000000005 | 10 | Alex | null |
ULTIPA800000000000000B | 7 | null |
null |
Insert Multiple Edges
insert().into(@follow).edges([
{_from_uuid: 1, _to_uuid: 2},
{_uuid: 9, _from: "U004", _to: "U003", time: "2023-9-10"},
{_from: "U002", _to: "U003"}
])
Three new edges are inserted with the following properties:
_uuid | _from | _to | _from_uuid |
_to_uuid | time |
---|---|---|---|---|---|
5 | U001 | U002 | 1 | 2 | null |
9 | U004 | U003 | 4 | 3 | 2023-09-10 00:00:00 |
6 | U002 | U003 | 2 | 3 | null |
Return Inserted Data
insert().into(@user).nodes([
{_id: "U006", name: "Joy"},
{_id: "U007", age: 41}
]) as n
return n{*}
Result:
_id | _uuid | name | age |
---|---|---|---|
U006 | 8 | Joy | null |
U007 | 9 | null |
41 |
Provide Point-Type Value
Use the point() function to specify the value of a point-type property.
insert().into(@city).nodes([
{ location: point({latitude: 132.1, longitude: -1.5}) }
])
Provide Blob-Type Value
Use the castToRaw() function to specify the value of a blob-type property.
insert().into(@city).nodes([
{profile_img: castToRaw("data:image/png;base64,iVBO0KGf8/9hAHNCSVQI=")}
])
Provide List-Type Value
Provide a list with elements enclosed in [ ]
and separated with ,
. The data type of each element should correspond to the type of the list.
Here's an example of inserting a node while providing the value for a list-type (float[]
) property ratings
:
insert().into(@city).nodes([
{ratings: [3.2, 6.7, 5.6, 5.6]}
])
Provide Set-Type Value
Provide a set with elements enclosed in [ ]
and separated with ,
. The data type of each element should correspond to the type of the set.
Here's an example of inserting a node while providing the value for a set-type (set(string)
) property tags
:
insert().into(@city).nodes([
{tags: ["hot", "art", "food"]}
])
Common Reasons for Failures
- Insertion fails when any provided values of unique identifier properties (
_id
,_uuid
) already exist in the graph. - Edge insertion fails when the specified start node or end node does not exist in the graph.
- Edge insertion fails when the start node or end node is not specified.